Blockchain Foundations: 1
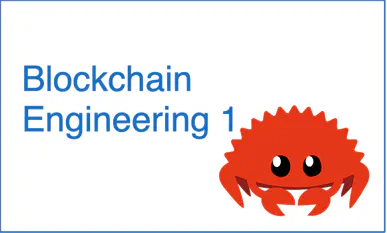
Table of Contents
What is a blockchain
Technical definition
A blockchain is a distributed ledger with growing lists of records (blocks) that are securely linked together via cryptographic hashes .[1] [2] [3] [4] Each block contains a cryptographic hash of the previous block, a timestamp , and transaction data (generally represented as a Merkle tree , where data nodes are represented by leaves). Since each block contains information about the previous block, they effectively form a chain (compare linked list data structure), with each additional block linking to the ones before it. Consequently, blockchain transactions are irreversible in that, once they are recorded, the data in any given block cannot be altered retroactively without altering all subsequent blocks.
Blockchains are typically managed by a peer-to-peer (P2P) computer network for use as a public distributed ledger , where nodes collectively adhere to a consensus algorithm protocol to add and validate new transaction blocks. Although blockchain records are not unalterable, since blockchain forks are possible, blockchains may be considered secure by design and exemplify a distributed computing system with high Byzantine fault tolerance .[5]
A blockchain was created by a person (or group of people) using the name (or pseudonym ) Satoshi Nakamoto in 2008 to serve as the public distributed ledger for bitcoin cryptocurrency transactions, based on previous work by Stuart Haber , W. Scott Stornetta , and Dave Bayer .[6] The implementation of the blockchain within bitcoin made it the first digital currency to solve the double-spending problem without the need for a trusted authority or central server . The bitcoin design has inspired other applications[3] [2] and blockchains that are readable by the public and are widely used by cryptocurrencies . The blockchain may be considered a type of payment rail .[7]
Simplistic definition
A blockchain is a chain of blocks, where each block is connected in a parent-child (one-to-one) relationship — forming the chain.
Elements of a blockchain
The blocks have properties like the:
- block index (nonce: number occurring once, difficulty)
- current block hash
- previous block hash
- data (often about transactions)
- timestamp
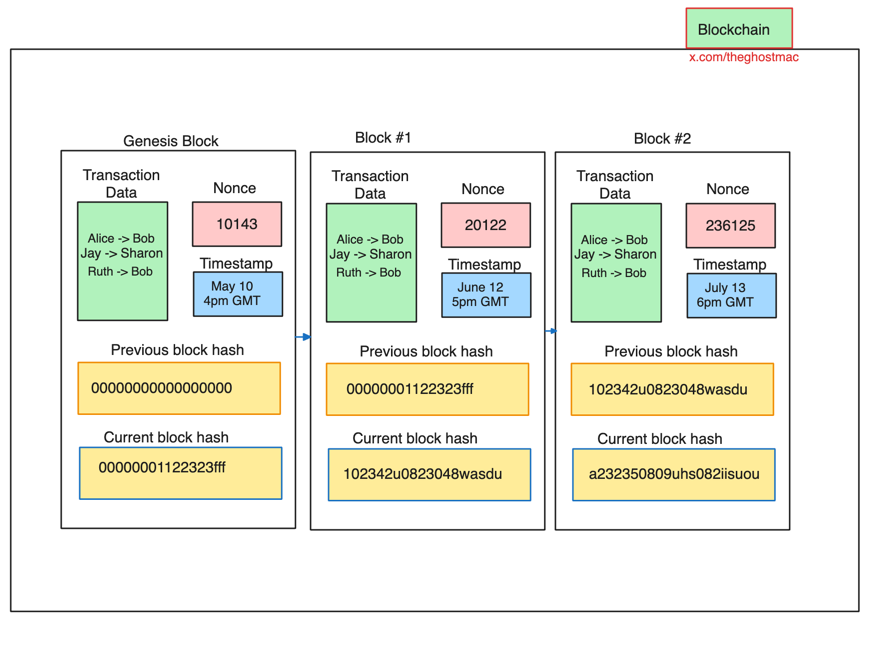
The first block has a set of unique properties given that it is actually the first block in the blockchain. Some of these properties include:
- No previous block hash: Since it’s the first block, there isn’t a preceding block to reference. This is a key difference from all subsequent blocks.
- Potential content: The first block, often called the Genesis Block, can contain additional information beyond the typical data field. This might include:
- Creation timestamp: This marks the official launch of the blockchain. For example, the Bitcoin Genesis Block was mined on January 3rd, 2009.
- Symbolic message: Some creators embed a message in the Genesis Block, perhaps signifying the purpose of the blockchain.
In essence, the Genesis Block acts as the foundation stone for the entire blockchain. It establishes the initial data structure and cryptographic hash reference point for all subsequent blocks.
The block header
The nonce/index, current block hash, previous block hash, and timestamp of a block makes up the block’s header. The block’s header is hashed using a cryptographic hash function, and this forms a correct “previous block hash”.
Implementing the current knowledge in Rust
First, we construct a block based on the elements in the block.
#[derive(Debug)]
pub struct Block {
index: u64,
block_hash: String,
previous_block_hash: String,
txn_data: String,
timestamp: u64,
}
Next, we create a constructor for a new block, with a dedicated hash derived from the block and the hash from the previous block.
impl Block {
pub fn new(index: u64, previous_block_hash: String, txn_data: String) -> Self {
let timestamp = SystemTime::now().duration_since(UNIX_EPOCH).unwrap().as_secs();
let block_hash = Self::calculate_hash(index, timestamp, &previous_block_hash, &txn_data);
// Construct a new Block.
Block {
index,
block_hash,
previous_block_hash,
txn_data,
timestamp
}
}
fn calculate_hash(index: u64, timestamp: u64, previous_block_hash: &str, txn_data: &str) -> String {
let to_hash = format!("{}:{}:{}:{}", index, timestamp, previous_block_hash, txn_data);
format!("{:x}", md5::compute(to_hash))
}
}
Afterwards, we initialize a new blockchain with a genesis block and a functionality to add subsequent blocks to it.
impl Blockchain {
pub fn new() -> Self {
let genesis_block = Block::new(0, "Genesis Block".to_string(), "".to_string());
Blockchain {
blocks: vec![genesis_block],
}
}
pub fn add_block(&mut self, txn_data: String) {
let previous_block = &self.blocks[self.blocks.len() -1];
let new_block = Block::new(
self.blocks.len() as u64,
previous_block.block_hash.clone(),
txn_data,
);
self.blocks.push(new_block);
}
}
We can run our dummy blockchain like this:
main.rs
:
mod block;
mod chain;
fn main() {
let mut blockchain = block::block_structure::Blockchain::new();
blockchain.add_block("Transaction 1".to_string());
blockchain.add_block("Transaction 2".to_string());
for block in blockchain.blocks {
println!("{:?}", block);
}
}
Conclusion
We made use of heuristic data types and simplistic implementations in this article to explain the elements of a blockchain. We will be improving on this in the next article, where we will use the actual data structures and more advanced implementations to further understand how blockchains truly work.
Related Posts
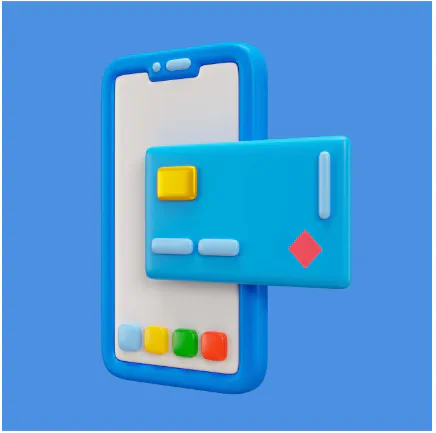
Simplifying Development with Docker Compose in Bankie.go
Simplifying Development with Docker Compose in Bankie.go Bankie.go , a simple banking application built with Go, has been updated to streamline the development and deployment processes using Docker Compose.
Read More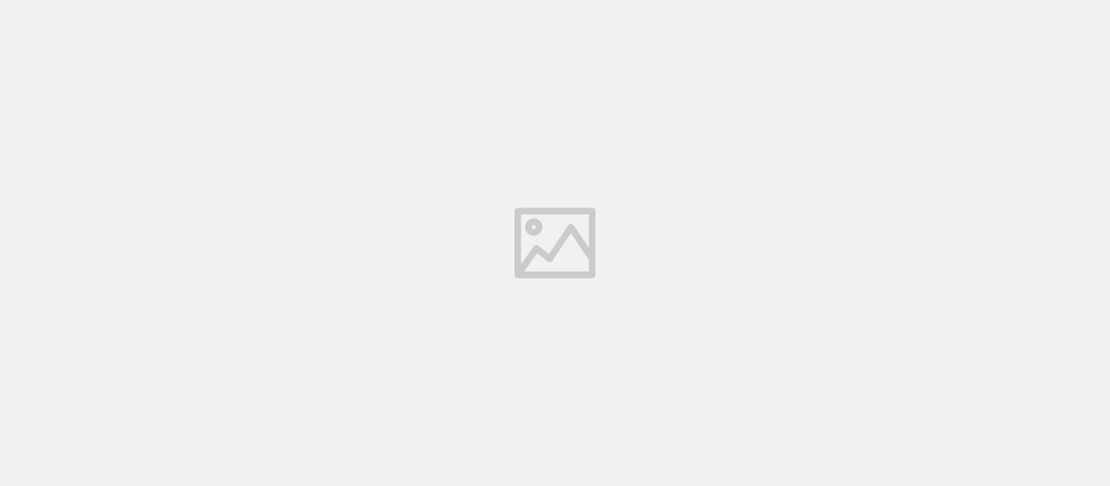
How to build an Application with modern Technology
Nemo vel ad consectetur namut rutrum ex, venenatis sollicitudin urna. Aliquam erat volutpat.
Read MoreUsing Merkle Trees for Token Gating in Blockchain DApps
Introduction Understanding Merkle Trees Merkle Trees, a concept named after Ralph Merkle who proposed it in 1979, stand as a cornerstone in cryptographic algorithms, especially within blockchain technology.
Read More